class: center, middle, inverse, title-slide # Intro to spatial analysis ### Jakub Nowosad
nowosad.jakub@gmail.com
### 2017-04-27 --- ## Introduction - R has powerful GIS capabilities due to many additional packages - see [CRAN Task View: Analysis of Spatial Data](https://cran.r-project.org/web/views/Spatial.html) and [CRAN Task View: Handling and Analyzing Spatio-Temporal Data](https://cran.r-project.org/web/views/SpatioTemporal.html) - **sf** and **sp** are the most important R packages to handle vector data; **sf** is a successor of **sp**, but it's still evolving. Moreover, many other R packages depend on the functions and classes for the **sp** package - **raster** is an extension of spatial data classes to work with rasters - there are many ways to vizualize spatial data in R, for example **ggplot2**, **rasterVis**, **tmap**, **leaflet**, and **mapview** packages - it is easy to connect R with a GIS software - GRASS GIS (**rgrass7**), SAGA (**RSAGA**), QGIS (**RQGIS**), and ArcGIS (**arcgisbinding**) --- class: inverse, center, middle ## Vector data --- ## The **sf** package The **sf** package in an R implementation of [Simple Features](https://en.wikipedia.org/wiki/Simple_Features). This package incorporates: - a new spatial data class system in R - functions for reading and writing data - tools for spatial operations on vectors Most of the functions in this package starts with prefix `st_`. ```r devtools::install_github('edzer/sfr') # development version ``` or ```r install.packages('sf') # stable version ``` You need a recent version of the GDAL, GEOS, Proj.4, and UDUNITS libraries installed for this to work on Mac and Linux. More information on that at https://github.com/edzer/sfr. ```r library('sf') ``` --- ## Reading spatial data ```r wrld <- st_read('data/wrld.shp') ``` ``` ## Reading layer `wrld' from data source `/home/jn/Documents/Intro_to_spatial_data/data/wrld.shp' using driver `ESRI Shapefile' ## converted into: POLYGON ## Simple feature collection with 177 features and 10 fields ## geometry type: MULTIPOLYGON ## dimension: XY ## bbox: xmin: -180 ymin: -90 xmax: 180 ymax: 83.64513 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ``` ```r ham <- st_read('data/hamilton_county.gpkg') ``` ``` ## Reading layer `hamilton_county.gpkg' from data source `/home/jn/Documents/Intro_to_spatial_data/data/hamilton_county.gpkg' using driver `GPKG' ## converted into: POLYGON ## Simple feature collection with 1 feature and 17 fields ## geometry type: POLYGON ## dimension: XY ## bbox: xmin: -84.8203 ymin: 39.02153 xmax: -84.25651 ymax: 39.31206 ## epsg (SRID): 4269 ## proj4string: +proj=longlat +ellps=GRS80 +towgs84=0,0,0,0,0,0,0 +no_defs ``` --- ## Reading spatial data - text files ```r ham_cities <- read.csv('data/hamiltion_cities.csv') ``` ```r ham_cities_sf <- st_as_sf(ham_cities, coords = c("X","Y")) ham_cities_sf ``` ``` ## Simple feature collection with 19 features and 2 fields ## geometry type: POINT ## dimension: XY ## bbox: xmin: -84.81995 ymin: 39.07862 xmax: -84.26384 ymax: 39.29034 ## epsg (SRID): NA ## proj4string: NA ## name place geometry ## 1 Delhi town POINT(-84.6052225 39.0950595) ## 2 Milford town POINT(-84.2958988 39.174625) ## 3 Covington town POINT(-84.508371 39.0836224) ## 4 Harrison town POINT(-84.8199516 39.2619993) ## 5 Cincinnati city POINT(-84.5124602 39.1014537) ## 6 Springdale town POINT(-84.4852213 39.287002) ## 7 Sycamore town POINT(-84.347427 39.2800883) ## 8 Norwood town POINT(-84.4596641 39.1556149) ## 9 Loveland town POINT(-84.2638406 39.2689562) ## 10 Fort Thomas town POINT(-84.4483432 39.0786242) ## 11 Saint Bernard town POINT(-84.4985541 39.1670033) ## 12 Montgomery town POINT(-84.3539662 39.2282432) ## 13 Forest Park town POINT(-84.5041108 39.2903353) ## 14 Blue Ash town POINT(-84.3782817 39.2320073) ## 15 Ludlow town POINT(-84.5474435 39.0925598) ## 16 Sharonville town POINT(-84.4132779 39.2681145) ## 17 Reading town POINT(-84.4421641 39.2236694) ## 18 Bellevue town POINT(-84.4826482 39.1067078) ## 19 Newport town POINT(-84.4919524 39.0889469) ``` --- ## Writing spatial data ```r st_write(wrld, 'data/new_wrld.shp') ``` ``` ## Writing layer `new_wrld' to data source `data/new_wrld.shp' using driver `ESRI Shapefile' ## features: 177 ## fields: 10 ## geometry type: Multi Polygon ``` ```r st_write(wrld, 'data/new_wrld.gpkg') ``` ``` ## Writing layer `new_wrld.gpkg' to data source `data/new_wrld.gpkg' using driver `GPKG' ## features: 177 ## fields: 10 ## geometry type: Multi Polygon ``` --- ## **sf** structure ```r class(wrld) ``` ``` ## [1] "sf" "data.frame" ``` **sf** objects usually have two classes - `sf` and `data.frame`. Two main differences comparing to a regular `data.frame` object are spatial metadata (`geometry type`, `dimension`, `bbox`, `epsg (SRID)`, `proj4string`) and additional column - typically named `geom` or `geometry`. ```r wrld[1:2, 1:3] ``` ``` ## Simple feature collection with 2 features and 3 fields ## geometry type: MULTIPOLYGON ## dimension: XY ## bbox: xmin: 11.6401 ymin: -17.93064 xmax: 75.15803 ymax: 38.48628 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ## iso_a2 name_long continent geometry ## 1 AF Afghanistan Asia MULTIPOLYGON(((61.210817091... ## 2 AO Angola Africa MULTIPOLYGON(((16.326528354... ``` --- ## Attributes - **sf** object can be used as a regular `data.frame` object in many operations ```r head(wrld) nrow(wrld) ncol(wrld) wrld[, c(1, 3)] wrld[1:5, 2] wrld[c(5, 10, 15), ] ``` - The `st_set_geometry` function can be used to remove the geometry column: ```r wrld_df <- st_set_geometry(wrld, NULL) class(wrld_df) ``` ``` ## [1] "data.frame" ``` --- ## Attributes - the **dplyr** package - It also easy to use the **dplyr** package on `sf` objects: ```r library('dplyr') ``` - `select()`: ```r wrld_sel <- select(wrld, name_long, area_km2) ``` ``` ## Simple feature collection with 4 features and 2 fields ## geometry type: MULTIPOLYGON ## dimension: XY ## bbox: xmin: 11.6401 ymin: -17.93064 xmax: 75.15803 ymax: 42.68825 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ## name_long area_km2 geometry ## 1 Afghanistan 652270.07 MULTIPOLYGON(((61.210817091... ## 2 Angola 1245463.75 MULTIPOLYGON(((16.326528354... ## 3 Albania 29694.80 MULTIPOLYGON(((20.590247430... ## 4 United Arab Emirates 79880.74 MULTIPOLYGON(((51.579518670... ``` --- ## Attributes - the **dplyr** package - `arrange()`: ```r wrld_arr <- arrange(wrld, area_km2) ``` ``` ## Simple feature collection with 3 features and 10 fields ## geometry type: MULTIPOLYGON ## dimension: XY ## bbox: xmin: 5.674052 ymin: 31.35344 xmax: 35.54567 ymax: 50.12805 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ## iso_a2 name_long continent region_un subregion ## 1 LU Luxembourg Europe Europe Western Europe ## 2 <NA> Northern Cyprus Asia Asia Western Asia ## 3 PS Palestine Asia Asia Western Asia ## type area_km2 pop lifeExp gdpPercap ## 1 Sovereign country 2416.870 556319 82.20732 90297.557 ## 2 Sovereign country 3786.365 NA NA NA ## 3 Disputed 5037.104 4294682 72.90402 4319.528 ## geometry ## 1 MULTIPOLYGON(((6.0430733577... ## 2 MULTIPOLYGON(((32.731780226... ## 3 MULTIPOLYGON(((35.545665317... ``` --- ## Attributes - the **dplyr** package - `filter()`: ```r wrld_fil <- filter(wrld, pop < 297517) ``` ``` ## Simple feature collection with 3 features and 10 fields ## geometry type: MULTIPOLYGON ## dimension: XY ## bbox: xmin: -73.297 ymin: -22.39998 xmax: 167.8449 ymax: 83.64513 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ## iso_a2 name_long continent region_un subregion ## 1 GL Greenland North America Americas Northern America ## 2 NC New Caledonia Oceania Oceania Melanesia ## 3 VU Vanuatu Oceania Oceania Melanesia ## type area_km2 pop lifeExp gdpPercap ## 1 Country 2206644.44 56295 NA NA ## 2 Dependency 23219.01 268000 77.57317 NA ## 3 Sovereign country 7490.04 258883 71.91832 2891.973 ## geometry ## 1 MULTIPOLYGON(((-46.76379 82... ## 2 MULTIPOLYGON(((165.77998986... ## 3 MULTIPOLYGON(((167.84487674... ``` --- ## Attributes - the **dplyr** package - `mutate()`: ```r wrld_mut <- mutate(wrld, pop_density = pop/area_km2) ``` ``` ## Simple feature collection with 3 features and 11 fields ## geometry type: MULTIPOLYGON ## dimension: XY ## bbox: xmin: 11.6401 ymin: -17.93064 xmax: 75.15803 ymax: 42.68825 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ## iso_a2 name_long continent region_un subregion type ## 1 AF Afghanistan Asia Asia Southern Asia Sovereign country ## 2 AO Angola Africa Africa Middle Africa Sovereign country ## 3 AL Albania Europe Europe Southern Europe Sovereign country ## area_km2 pop lifeExp gdpPercap pop_density ## 1 652270.1 31627506 60.37446 1844.022 48.48836 ## 2 1245463.7 24227524 52.26688 6955.960 19.45261 ## 3 29694.8 2893654 77.83046 10698.525 97.44649 ## geometry ## 1 MULTIPOLYGON(((61.210817091... ## 2 MULTIPOLYGON(((16.326528354... ## 3 MULTIPOLYGON(((20.590247430... ``` --- ## Attributes - the **dplyr** package - `summarize()`: ```r wrld_sum1 <- summarize(wrld, pop_sum = sum(pop, na.rm = TRUE), pop_mean = mean(pop, na.rm = TRUE), pop_median = median(pop, na.rm = TRUE)) ``` ``` ## pop_sum pop_mean pop_median ## 1 7208968708 42910528 10521458 ``` ```r wrld_sum1 <- wrld %>% group_by(continent) %>% summarize(pop_sum = sum(pop, na.rm = TRUE), pop_mean = mean(pop, na.rm = TRUE), pop_median = median(pop, na.rm = TRUE)) ``` ``` ## Simple feature collection with 3 features and 4 fields ## geometry type: GEOMETRY ## dimension: XY ## bbox: xmin: -180 ymin: -90 xmax: 180 ymax: 55.38525 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ## # A tibble: 3 × 5 ## continent pop_sum pop_mean pop_median geometry ## <fctr> <dbl> <dbl> <dbl> <simple_feature> ## 1 Africa 1147005839 23895955 14129805 <MULTIPOLYGON...> ## 2 Antarctica 0 NaN NA <MULTIPOLYGON...> ## 3 Asia 4306025131 95689447 18772481 <MULTIPOLYGON...> ``` --- ## CRS assign - In case when a coordinate reference system (CRS) is missing or the wrong CRS is set, `st_crs()` or `st_set_crs` function can be used: ```r wrld_set3410 <- st_set_crs(wrld, 3410) st_crs(wrld_set3410) ``` ``` ## $epsg ## [1] 3410 ## ## $proj4string ## [1] "+proj=cea +lon_0=0 +lat_ts=30 +x_0=0 +y_0=0 +a=6371228 +b=6371228 +units=m +no_defs" ## ## attr(,"class") ## [1] "crs" ``` 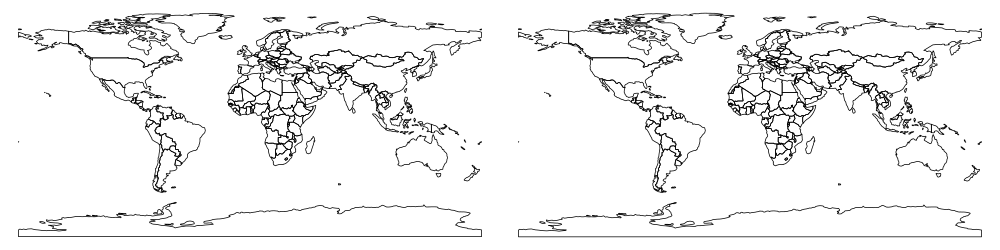 --- ## CRS assign ```r st_crs(ham_cities_sf) ``` ``` ## $epsg ## [1] NA ## ## $proj4string ## [1] NA ## ## attr(,"class") ## [1] "crs" ``` ```r ham_cities_sf <- st_set_crs(ham_cities_sf, 4326) st_crs(ham_cities_sf) ``` ``` ## $epsg ## [1] 4326 ## ## $proj4string ## [1] "+proj=longlat +datum=WGS84 +no_defs" ## ## attr(,"class") ## [1] "crs" ``` --- ## Reprojection - The `st_transform()` can be used to transform coordinates ```r wrld_3410 <- st_transform(wrld, 3410) st_crs(wrld_3410) ``` ``` ## $epsg ## [1] 3410 ## ## $proj4string ## [1] "+proj=cea +lon_0=0 +lat_ts=30 +x_0=0 +y_0=0 +a=6371228 +b=6371228 +units=m +no_defs" ## ## attr(,"class") ## [1] "crs" ``` 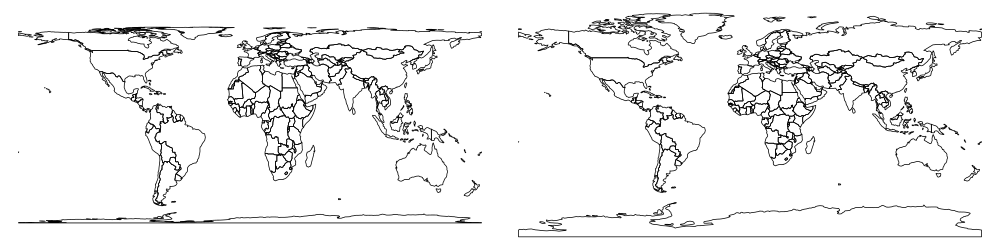 --- ## Basic maps - Basic maps of `sf` object can be quickly created using the `plot()` function: ```r plot(wrld[0]) ``` ```r plot(wrld["pop"]) ``` 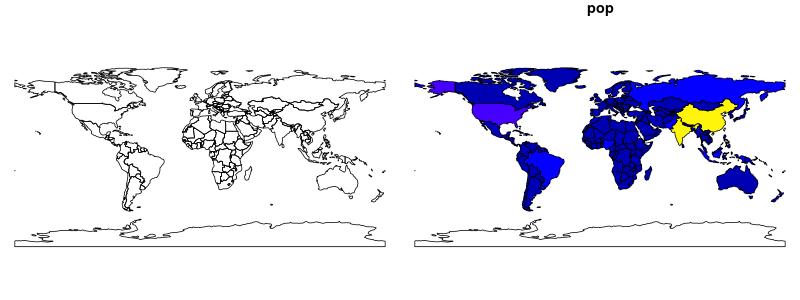 --- ## The **sp** package - the **sp** package is a predecessor of the **sf** package - together with the **rgdal** and **rgeos** package it creates a powerful tool to works with spatial data - many spatial R packages still depends on the **sp** package, therefore it is important to know how to convert **sp** to and from **sf** objects ```r wrld_sp <- as(wrld, 'Spatial') class(wrld_sp) ``` ``` ## [1] "SpatialPolygonsDataFrame" ## attr(,"package") ## [1] "sp" ``` ```r wrld_sf <- st_as_sf(wrld_sp) class(wrld_sf) ``` ``` ## [1] "sf" "data.frame" ``` <!-- predesessor of sf--> <!-- rgdal, rgeos--> <!-- still used by many packages--> <!-- how to convert (both ways)--> --- class: inverse, center, middle ## Raster data --- ## The **raster** package The **raster** package consists of method and classes for raster processing. It allows to: - read and write raster data - perform raster algebra and raster manipulations - work on large datasets due to its ability to process data in chunks - visualize raster data - many more... This package has three object classes: - `RasterLayer` - for single-layer objects - `RasterStack` - for multi-layer objects from separate files or a few layers from a single file - `RasterBrick` - for multi-layer objects linked to a single file <!-- raster info --> <!-- old timer--> <!-- three types of data --> ```r library('raster') ``` --- ## Reading ```r dem <- raster('data/srtm.tif') dem ``` ``` ## class : RasterLayer ## dimensions : 720, 1200, 864000 (nrow, ncol, ncell) ## resolution : 0.0008333333, 0.0008333333 (x, y) ## extent : -85.00042, -84.00042, 38.79958, 39.39958 (xmin, xmax, ymin, ymax) ## coord. ref. : +proj=longlat +datum=WGS84 +no_defs +ellps=WGS84 +towgs84=0,0,0 ## data source : /home/jn/Documents/Intro_to_spatial_data/data/srtm.tif ## names : srtm ## values : 109, 323 (min, max) ``` --- ## Writing ```r writeRaster(dem, 'data/new_dem.tif') ``` ```r writeRaster(dem, 'data/new_dem2.tif', datatype = 'FLT4S', options=c("COMPRESS=DEFLATE")) ``` ```r writeFormats() ``` ``` ## name long_name ## [1,] "raster" "R-raster" ## [2,] "SAGA" "SAGA GIS" ## [3,] "IDRISI" "IDRISI" ## [4,] "IDRISIold" "IDRISI (img/doc)" ## [5,] "BIL" "Band by Line" ## [6,] "BSQ" "Band Sequential" ## [7,] "BIP" "Band by Pixel" ## [8,] "ascii" "Arc ASCII" ## [9,] "CDF" "NetCDF" ## [10,] "big" "big.matrix" ## [11,] "ADRG" "ARC Digitized Raster Graphics" ## [12,] "BMP" "MS Windows Device Independent Bitmap" ## [13,] "BT" "VTP .bt (Binary Terrain) 1.3 Format" ## [14,] "CTable2" "CTable2 Datum Grid Shift" ## [15,] "EHdr" "ESRI .hdr Labelled" ## [16,] "ELAS" "ELAS" ## [17,] "ENVI" "ENVI .hdr Labelled" ## [18,] "ERS" "ERMapper .ers Labelled" ## [19,] "FITS" "Flexible Image Transport System" ## [20,] "GPKG" "GeoPackage" ## [21,] "GS7BG" "Golden Software 7 Binary Grid (.grd)" ## [22,] "GSBG" "Golden Software Binary Grid (.grd)" ## [23,] "GTiff" "GeoTIFF" ## [24,] "GTX" "NOAA Vertical Datum .GTX" ## [25,] "HDF4Image" "HDF4 Dataset" ## [26,] "HFA" "Erdas Imagine Images (.img)" ## [27,] "IDA" "Image Data and Analysis" ## [28,] "ILWIS" "ILWIS Raster Map" ## [29,] "INGR" "Intergraph Raster" ## [30,] "ISCE" "ISCE raster" ## [31,] "ISIS2" "USGS Astrogeology ISIS cube (Version 2)" ## [32,] "KRO" "KOLOR Raw" ## [33,] "LAN" "Erdas .LAN/.GIS" ## [34,] "Leveller" "Leveller heightfield" ## [35,] "MBTiles" "MBTiles" ## [36,] "MRF" "Meta Raster Format" ## [37,] "netCDF" "Network Common Data Format" ## [38,] "NITF" "National Imagery Transmission Format" ## [39,] "NTv2" "NTv2 Datum Grid Shift" ## [40,] "PAux" "PCI .aux Labelled" ## [41,] "PCIDSK" "PCIDSK Database File" ## [42,] "PCRaster" "PCRaster Raster File" ## [43,] "PDF" "Geospatial PDF" ## [44,] "PNM" "Portable Pixmap Format (netpbm)" ## [45,] "RMF" "Raster Matrix Format" ## [46,] "ROI_PAC" "ROI_PAC raster" ## [47,] "RST" "Idrisi Raster A.1" ## [48,] "SAGA" "SAGA GIS Binary Grid (.sdat)" ## [49,] "SGI" "SGI Image File Format 1.0" ## [50,] "Terragen" "Terragen heightfield" ``` --- ## **raster** structure ```r dem ``` ``` ## class : RasterLayer ## dimensions : 720, 1200, 864000 (nrow, ncol, ncell) ## resolution : 0.0008333333, 0.0008333333 (x, y) ## extent : -85.00042, -84.00042, 38.79958, 39.39958 (xmin, xmax, ymin, ymax) ## coord. ref. : +proj=longlat +datum=WGS84 +no_defs +ellps=WGS84 +towgs84=0,0,0 ## data source : /home/jn/Documents/Intro_to_spatial_data/data/srtm.tif ## names : srtm ## values : 109, 323 (min, max) ``` ```r inMemory(dem) ``` ``` ## [1] FALSE ``` --- ## Attributes - the `getValues` function returns values from a raster object: ```r values_dem <- getValues(dem) ``` ``` ## [1] 191 194 202 204 198 193 189 186 187 188 192 206 221 229 236 238 241 ## [18] 236 226 217 209 205 201 198 203 211 218 220 225 232 237 236 238 242 ## [35] 247 254 257 261 260 258 264 270 272 276 280 281 276 266 266 270 ``` - it is possible to specify a number of rows to extract its values: ```r getValues(dem, row = 5) ``` - there are two additional functions - `getValuesBlock` (to get a block of raster values) and `getValuesFocal` (to get focal raster cell values) --- ## Attributes .pull-left[ ```r new_values <- runif(864000, min=150, max=300) # pseudo-random number generator new_dem <- setValues(dem, new_values) ``` <img src="Intro_to_spatial_analysis_files/figure-html/unnamed-chunk-53-1.png" style="display: block; margin: auto;" /> ] .pull-right[ ```r new_dem2 <- dem new_dem2[new_dem2 < 0] <- NA ``` <img src="Intro_to_spatial_analysis_files/figure-html/unnamed-chunk-55-1.png" style="display: block; margin: auto;" /> ] --- ## Attributes .pull-left[ ```r new_dem3 <- dem + 50 ``` <img src="Intro_to_spatial_analysis_files/figure-html/unnamed-chunk-57-1.png" style="display: block; margin: auto;" /> ] .pull-right[ ```r new_dem4 <- dem * new_dem ``` <img src="Intro_to_spatial_analysis_files/figure-html/unnamed-chunk-59-1.png" style="display: block; margin: auto;" /> ] --- ## CRS assign ```r dem_set3410 <- dem crs(dem_set3410) <- "+proj=cea +lon_0=0 +lat_ts=30 +x_0=0 +y_0=0 +a=6371228 +b=6371228 +units=m +no_defs" ``` ```r crs(dem_set3410) ``` ``` ## CRS arguments: ## +proj=cea +lon_0=0 +lat_ts=30 +x_0=0 +y_0=0 +a=6371228 +b=6371228 ## +units=m +no_defs ``` --- ## Reprojection ```r dem3410 <- projectRaster(dem, crs="+proj=cea +lon_0=0 +lat_ts=30 +x_0=0 +y_0=0 +a=6371228 +b=6371228 +units=m +no_defs") ``` 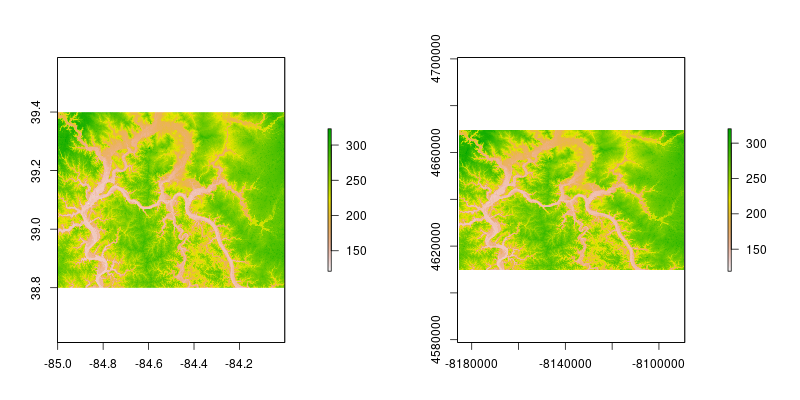 --- ## Simple map ```r plot(dem) ``` <img src="Intro_to_spatial_analysis_files/figure-html/unnamed-chunk-64-1.png" style="display: block; margin: auto;" /> --- class: inverse, center, middle ## Vector-Raster interactions --- ## Extract ```r ham_cities_sp <- as(ham_cities_sf, 'Spatial') raster::extract(dem, ham_cities_sp) ``` ``` ## [1] 276 160 157 161 188 226 260 202 184 261 171 243 258 260 165 178 173 ## [18] 162 162 ``` ```r ham_cities_sf$dem <- raster::extract(dem, ham_cities_sp) ham_cities_sf ``` ``` ## Simple feature collection with 19 features and 3 fields ## geometry type: POINT ## dimension: XY ## bbox: xmin: -84.81995 ymin: 39.07862 xmax: -84.26384 ymax: 39.29034 ## epsg (SRID): 4326 ## proj4string: +proj=longlat +datum=WGS84 +no_defs ## name place geometry dem ## 1 Delhi town POINT(-84.6052225 39.0950595) 276 ## 2 Milford town POINT(-84.2958988 39.174625) 160 ## 3 Covington town POINT(-84.508371 39.0836224) 157 ## 4 Harrison town POINT(-84.8199516 39.2619993) 161 ## 5 Cincinnati city POINT(-84.5124602 39.1014537) 188 ## 6 Springdale town POINT(-84.4852213 39.287002) 226 ## 7 Sycamore town POINT(-84.347427 39.2800883) 260 ## 8 Norwood town POINT(-84.4596641 39.1556149) 202 ## 9 Loveland town POINT(-84.2638406 39.2689562) 184 ## 10 Fort Thomas town POINT(-84.4483432 39.0786242) 261 ## 11 Saint Bernard town POINT(-84.4985541 39.1670033) 171 ## 12 Montgomery town POINT(-84.3539662 39.2282432) 243 ## 13 Forest Park town POINT(-84.5041108 39.2903353) 258 ## 14 Blue Ash town POINT(-84.3782817 39.2320073) 260 ## 15 Ludlow town POINT(-84.5474435 39.0925598) 165 ## 16 Sharonville town POINT(-84.4132779 39.2681145) 178 ## 17 Reading town POINT(-84.4421641 39.2236694) 173 ## 18 Bellevue town POINT(-84.4826482 39.1067078) 162 ## 19 Newport town POINT(-84.4919524 39.0889469) 162 ``` --- ## Crop ```r ham84 <- st_transform(ham, 4326) ham_sp <- as(ham84, 'Spatial') ``` .pull-left[ ```r dem_crop <- crop(dem, ham_sp) ``` 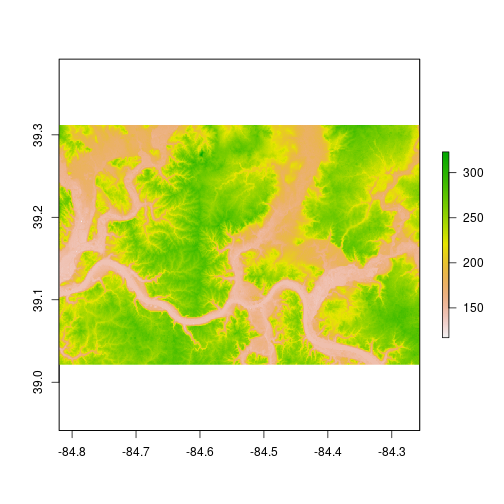<!-- --> ] .pull-right[ ```r dem_mask <- mask(dem_crop, ham_sp) ``` 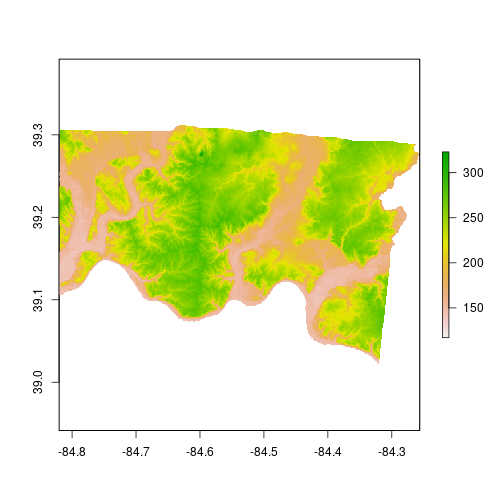<!-- --> ] --- class: inverse, center, middle ## Advanced map-making --- ## rasterVis - https://oscarperpinan.github.io/rastervis/ - http://www.colorbrewer.org ```r library('rasterVis') my_theme <- rasterTheme(region=brewer.pal('RdYlGn', n = 9)) p <- levelplot(dem_crop, margin = FALSE, par.settings = my_theme) p <- p + layer(sp.lines(ham_sp, lwd = 3, col = 'darkgrey')) p + layer(sp.points(ham_cities_sp, pch = 19, col = 'black')) ``` <img src="Intro_to_spatial_analysis_files/figure-html/unnamed-chunk-73-1.png" style="display: block; margin: auto;" /> --- ## tmap - https://cran.r-project.org/web/packages/tmap/vignettes/tmap-nutshell.html ```r library('tmap') tm_shape(wrld, projection="wintri") + tm_polygons("lifeExp", style="pretty", palette="RdYlGn", auto.palette.mapping=FALSE, title=c("Life expactancy")) + tm_style_grey() ``` <img src="Intro_to_spatial_analysis_files/figure-html/unnamed-chunk-74-1.png" style="display: block; margin: auto;" /> --- ## leaflet ```r library('leaflet') leaflet(ham_sp) %>% addProviderTiles(providers$Stamen.Watercolor) %>% # addTiles() %>% addPolygons() %>% addMarkers(data=ham_cities_sp, popup=~as.character(name)) ```
--- class: inverse, center, middle ## Spatial data sources --- ## Spatial data sources - [The osmdata package](https://github.com/osmdatar/osmdata) - for downloading OpenStreetMap data - [The tigirs package](https://github.com/walkerke/tigris) - Census TIGER/Line shapefiles in R - [The USAboundaries package](https://github.com/ropensci/usaboundaries/) - contemporary state, county, and congressional district boundaries for the United States of America, as well as historical boundaries from 1629 to 2000 for states and counties - [The rnaturalearth package](https://github.com/ropenscilabs/rnaturalearth) - Natural Earth map data - [The MODIStsp package](https://github.com/lbusett/MODIStsp) - automatic download and preprocessing of MODIS Land Products Time Series - [The rWBclimate](https://github.com/ropensci/rWBclimate) - the World Bank climate data - [The GSODR package](https://github.com/ropensci/GSODR) - Global Summary Daily Weather Data - [The rnoaa package](https://github.com/ropensci/rnoaa/) - an R interface to many NOAA data sources - [The hddtools package](https://github.com/ropensci/hddtools/) - Hydrological Data Discovery Tools - [The spData package](https://github.com/Nowosad/spData) - datasets for spatial analysis --- class: inverse, center, middle ## Resources --- ## Resources - [sf vignette](https://edzer.github.io/sfr/articles/) - documentation of the **sf** package - [HOW-TO: Mapping in R just got a whole lot easier](http://www.computerworld.com/article/3175623/data-analytics/mapping-in-r-just-got-a-whole-lot-easier.html) - an introduction to mapping using the sf package - [Spatial analysis in R with the sf package](https://cdn.rawgit.com/rhodyrstats/geospatial_with_sf/bc2b17cf/geospatial_with_sf.html) - an introduction to spatial analysis using the sf package - [Tidy spatial data in R: using dplyr, tidyr, and ggplot2 with sf](http://strimas.com/r/tidy-sf/) - [Introduction to Working With Raster Data in R](http://neondataskills.org/tutorial-series/raster-data-series/) - a series of introductory tutorials to the **raster** package - [Map and analyze raster data in R](http://zevross.com/blog/2015/03/30/map-and-analyze-raster-data-in-r/) - examples of how to work with raster data in R - [The Visual Raster Cheat Sheet](http://rpubs.com/etiennebr/visualraster) - great illustrations of some operations of the **raster** package - [Introduction to rasterVis](https://rpubs.com/alobo/rasterVis_Intro1) - [Leaflet for R](http://rstudio.github.io/leaflet/) - documentation of the **leaflet** package - [Spatial Data Analysis and Modeling with R](http://rspatial.org/index.html) - materials to learn about spatial data analysis and modeling with R - [Creating-maps-in-R](https://github.com/Robinlovelace/Creating-maps-in-R) - introductory tutorial on graphical display of geographical information in R - [Downloading and plotting MODIS Chlorophyll-a data](http://clarkrichards.org/r/oce/modis/chl/sp/raster/2017/03/25/modis-chl-data/) <!-- our book?? -->